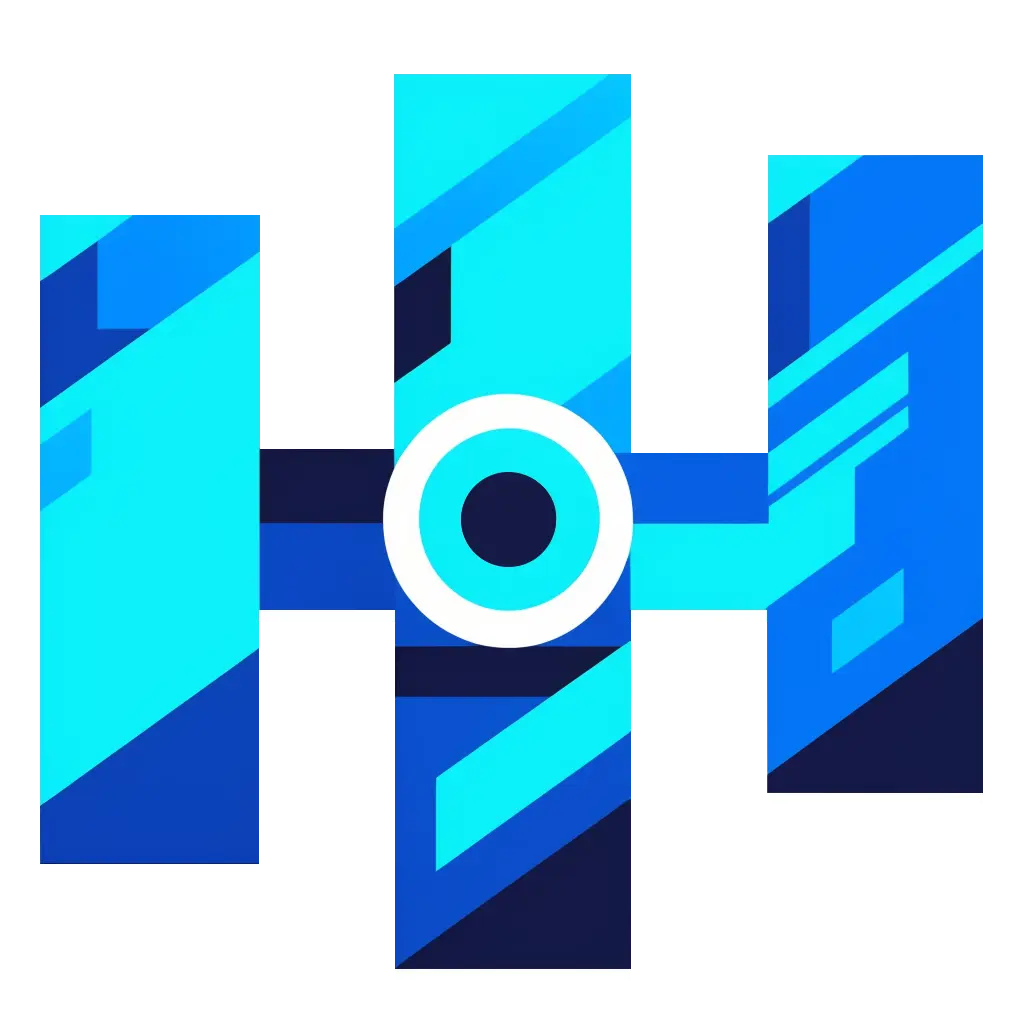
Menter
A functional programming language written in Java.Menter is a programming language that is built in Java, meaning that it can be run on any platform that supports Java. It has a strong emphasis on simplicity and ease of use.
This collection of pages is a guide and documentation to the Menter programming language. Start with the introduction of the Menter Guide to get a quick overview of the language and its features.
A Quick Sample
Here's a small program that creates an array, maps them to new values and filters them:
Main Features
Functional programming
Menter is a functional programming language, meaning that functions are first class citizens and can be passed around like any other value. It also supports higher order functions, meaning that functions can be passed to other functions and functions can return functions.
Useful operators
Menter takes the regular operators that most languages have and adds some exiting new feature on top:
Object oriented programming
Menter also supports object-oriented programming. Custom type definitions are represented by
functions that return a new object. These functions can be called with the new
keyword.
Module concept
To allow for multi-file projects, Menter supports modules. Modules can export symbols, which can then be imported by other modules. This allows for a clean separation of code and makes it easy to reuse code.
Extensibility via Java
Menter is designed to be easily extensible via Java. You can add new functions, operators, types and more. Learn more about this in the Java section.
But, what is this language?
The main reason this language exists is to provide a simple, but powerful expression evaluator for the Launch Anything project. It is designed to be easy to use and to be able to be embedded into other projects.
Another reason is, that I have always been interested in compilers/interpreters and wanted to
finally try and dip my toes into the topic by creating a simple programming language.
Seeing as I mainly am a Java developer, I decided to write it in Java. This was also supported by
the fact that the Launch Anything project is also written in Java.
But to speak in buzzwords, Menter is a:
functional, single threaded, dynamically typed, interpreted, object oriented, garbage collected,
statically scoped, expression based, programming language, that can be extended and adjusted for
your needs with Java code.
Got you hooked? Get started with the introduction of the Menter Guide to get a quick overview of the language and its features.
© 2023 Yan Wittmann